1.1 异常的概念
异常指的是运行期出现的错误,也就是当程序开始执行以后执行期出现的错误。出现错误时观察错误的名字和行号最为重要。
在这个世界不可能存在完美的东西,不管完美的思维有多么缜密,细心,我们都不可能考虑所有的因素,这就是所谓的智者千虑必有一失。同样的道理,计算机的世界也是不完美的,异常情况随时都会发生,我们所需要做的就是避免那些能够避免的异常,处理那些不能避免的异常。这里我将记录如何利用异常还程序一个“完美世界”。
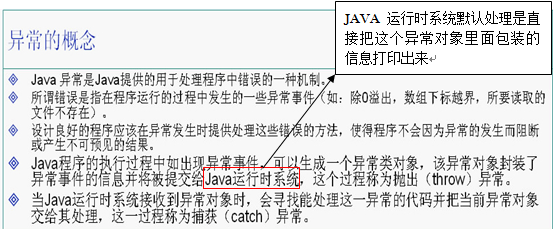
异常情况例如:
- 工厂生产,原料用尽
- 高速路上车没油
- 家里灯泡断电
1.2 异常分类
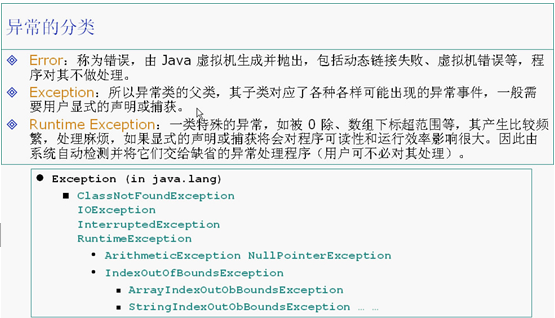
错误:
错误不是异常,是用户和程序无法控制的问题,这是系统内部的错误。
检查异常:
检查异常通常是用户错误或者不能被程序所预见的问题,例如,打开一个文件,但是文件不存在,这中错误称为检查异常,必须由java语言来处理。
运行时异常:
运行时异常是程序在运行过程中可能发生的,可以被程序员避免的异常,可以被忽略,提示我们开发人员进行处理。
1.3 异常的控制流程
首先来看一段代码:
上面的三个方法都被押入到内存的方法栈中,如果说某一段代码出现了异常那代码会如何执行呢?
在method2中加入了 int n = 10/0;
程序运行到这句话是肯定要抛出异常。那我们会得到什么结果呢?
|
|
- main方法在调用栈的最底部,method2在最顶部,如果method2抛出一个异常,method2就会从栈中被取出,
- 同时将异常继续抛给调用他的method1方法,发现method1并没有处理这个异常,那直接抛给main方法,并从栈中退出。
- 这时main方法方法也没有处理异常,就有java虚拟机来处理这个异常,虚拟机会创建一个exception对象将信息打印到控制台,然后结束程序。
通过画图来分析异常抛出的流程!
1.4 异常的体系结构
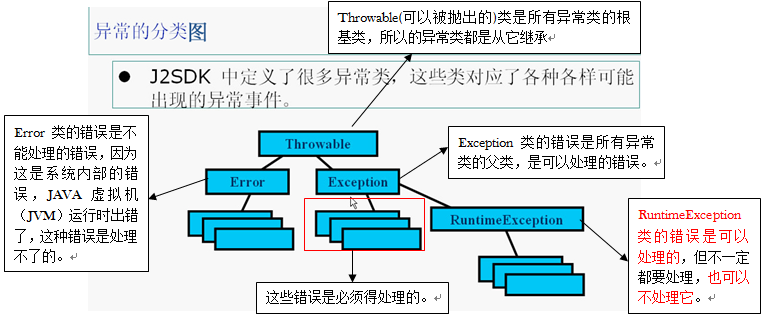
通过api来进行异常类的查看和分析。
1.5 捕获异常
在网上看了这样一个搞笑的话:世界上最真情的相依,是你在try我在catch。无论你发神马脾气,我都默默承受,静静处理。对于初学者来说异常就是try…catch
如果我们发现程序某段代码会抛出异常,那我们要去捕获这个异常。
Java异常处理的五个关键字:try、catch、finally、throw、throws
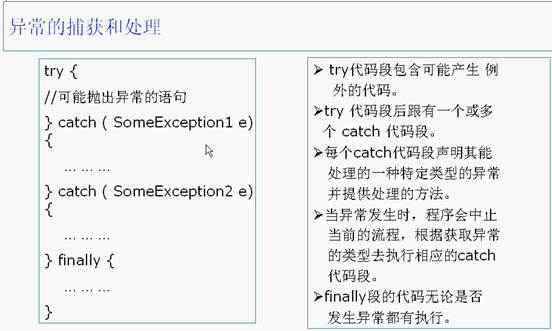
例子:
多个catch块使用
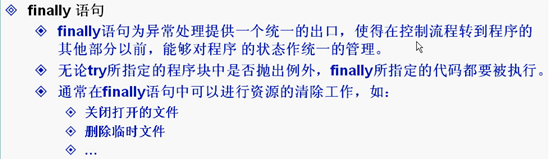
1.6 申明并抛出异常
1.6.1 throw和throws
throw:将异常进行抛出(动作)
throws:声明将要抛出何种类型的异常(声明)
例子:
|
|
在method方法里面就必须要处理这个异常
|
|
1.6.2 重写方法异常
- 父类中未抛出异常
创建一个parent父类
|
|
子类继承没有抛出异常的父类
|
|
结果:编译不通过。IDE告诉我们“overridden method does not throw ‘java.lang.Exception’”,好尴尬不是么!不过还好,我们吸取了教训,知道了父类未抛出异常时,子类也不能抛出异常的约定,至少没在项目发布上线后才发现问题,万幸万幸。
- 父类中抛出异常
|
|
同样的,再搞一个子类出来,或者也可以将上面的子类稍作修改。如下:
|
|
|
|
如果…我子类不是抛出Exception,而是其子类比如IOException呢?
|
|
child test
那我把子类和父类的异常掉个包应该也没问题吧:
|
|
|
|