打印万年历,你会吗?啊,不会!那就跟我一起使用Java在控制台上打印一个简单的万年历吧!
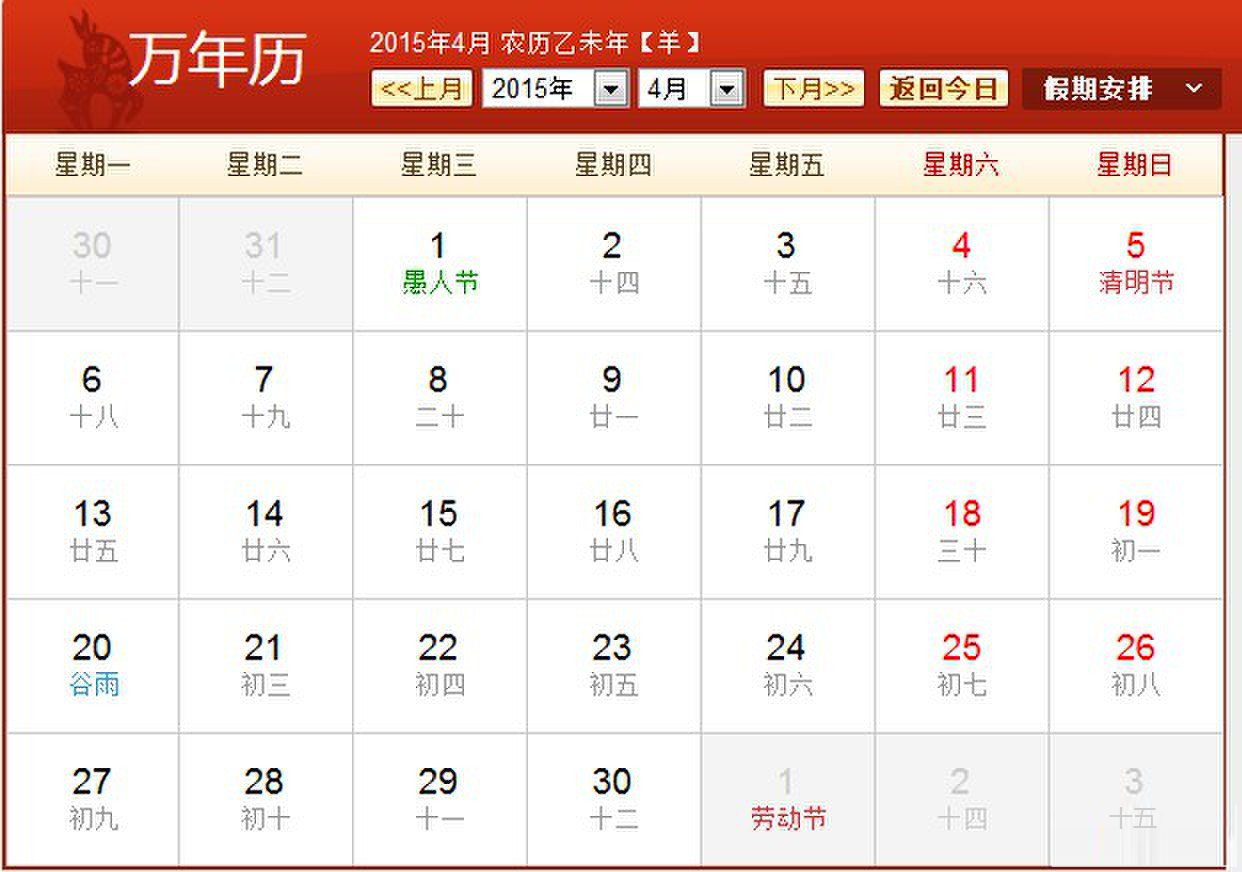
这个万年历怎么样,漂亮吧!
你该不会天真的认为这是从控制台上打印出来吧?要是你真这么想,我可被你的天真打败了!
要用Java实现这个万年历不是不可能,你还得会Java的swing图形用户界面开发。
我们还是来看看Java在控制台上打印的万年历长啥样?
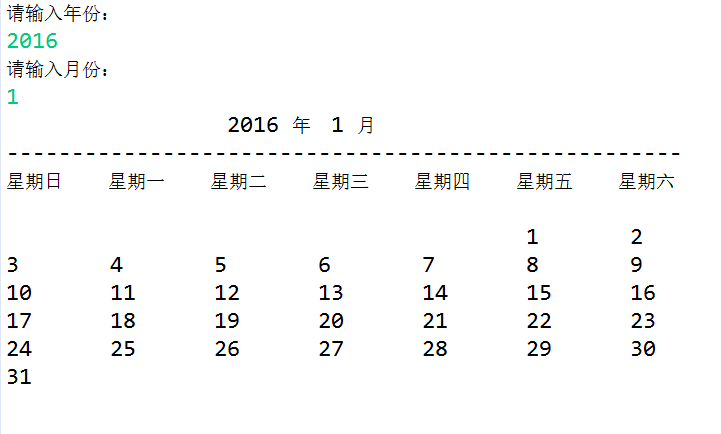
第一反应是不是好丑呀?别看它长得丑,这可是货真价实的万年历。
软件开发其实就好比一个人,最重要的是内涵(功能),而不是外表(界面)(其实外表也很重要)。这个万年历就很有内涵。不信,我们就一起来看一看如何实现万年历吧。
初学编程的同学在写代码时,习惯还没想好就开始写代码,写的过程中有问题又反复修改。如果是语文考试作文,恐怕你的作文最终已经改得面目全非,惨不忍睹。所以,这是一个非常不好的编程习惯。
好的编程习惯是解决问题前一定要先分析问题,把大问题逐步分成小问题,理清逻辑,自下而上,逐步实现。
其实,一句话就是先想好再动手。
问题分析
首先1970年是Unix系统诞生的时间,1970年就成为Unix的元年,1970年1月1号是星期四,现在大多的手机的日历功能只能显示到1970年1月1日这一天。不信,现在赶紧掏出手机看一看。那最大可以显示哪一年呢?我的手机显示的是2037年,你的呢?
要想打印某年某月的日历,逐步分成小问题如下:
1> 计算出本月的总天数 (注意:考虑闰年问题)
2> 计算出本月的1号是星期几(其他号数对应的星期几就可以以此类推)
打印万年历格式
1>打印标题格式
2>打印内容格式
代码实现
问题分析得差不多了,心里应该大致知道要实现哪些小功能,接着就一步步编写代码。
Java的JDK帮助文档中,有一个专门日历类-Calender。这个类封装了很多方法用于计算日历,我们正好使用它来完成万年历的开发。
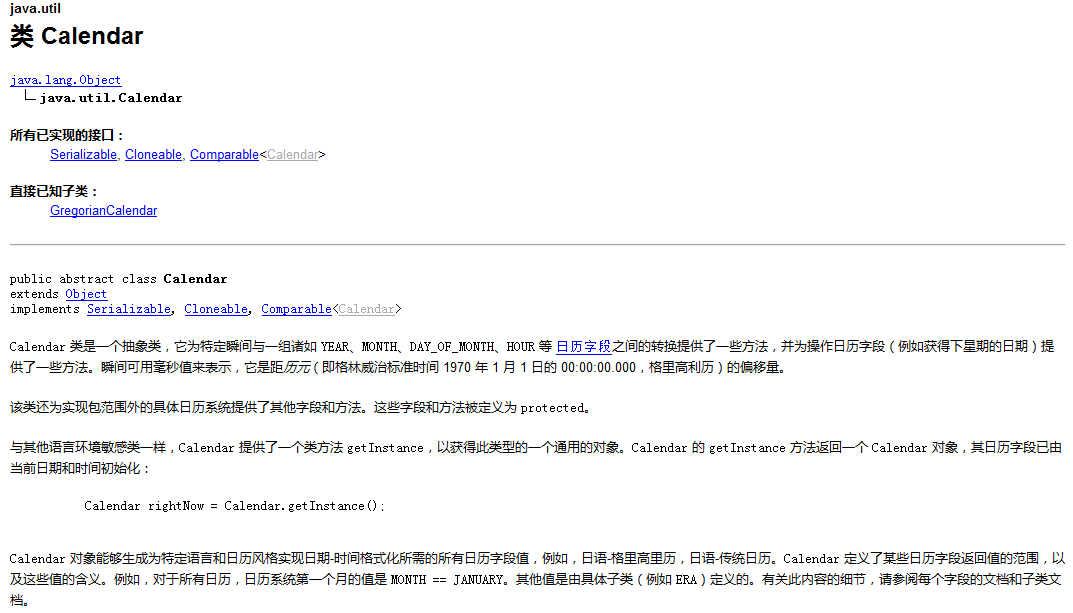
下面写一个Calender简单小例子:
|
|
输出结果如下(2017年6月9日 星期五):
2017
5
9
6
Calendar类在实现万年历中的具体使用,我们通过代码进行说明,方法的使用有不清楚的地方可以自行查看JDK帮助文档。
- 判断是否闰年方法
|
|
- 计算本月天数
|
|
- 计算本月第一天星期几
|
|
- 打印标题
|
|
- 打印内容
|
|
- 打印万年历
|
|
总结
你现在觉得打印这个简单的万年历其实并不简单,很有内涵了吧?涉及很多编程的细节和小问题的处理。是不是感觉一下子脑袋又不够用了?
还等什么,现在就跟着上面的代码,自己再敲一遍。边敲那思考,直到自己搞明白为此。